Sep 28, 2023 By Team YoungWonks *
What is JavaScript or JS?
JavaScript, often abbreviated as JS, is a high-level, interpreted programming language that is one of the core technologies of the World Wide Web. This versatile language is used to make web pages interactive and create online programs, including video games. It is an essential skill for web developers to create dynamic and interactive web applications. Despite its name, JavaScript has no direct relation to Java, and it is characterized by its flexibility and ease of use, making it a good starting point for those new to programming. For more information, please read Java vs JavaScript blog.
What is a string in JavaScript?
In JavaScript, a string is a primitive data type that represents a sequence of characters. Strings in JavaScript are used to store and manipulate text-based information within a program. They are created by enclosing text within single quotes (' '), double quotes (" "), or backticks (` `). For example, 'Hello there!', "YoungWonks Coding Classes", and `Python Programming` are all valid strings in JavaScript. Strings can be manipulated using methods provided by JavaScript's built-in String object, allowing programmers to perform operations such as slicing, splitting, replacing, and many others.
JavaScript String Format Techniques
JavaScript, in web development, offers a variety of methods for string formatting. These methods make it easier to structure and manipulate text data in your applications. This tutorial aims to provide a comprehensive overview of JavaScript string format techniques, perfect for beginners and those looking to refresh their knowledge.
Let's start with the basics, using a simple string as our example. In JavaScript, a string is a sequence of characters enclosed in either single quotes, double quotes, or backticks. For instance, we could define a string, const message = 'Hello World!', and this message string could be displayed in a browser console using console.log(message).
String Concatenation
What does concatenation mean? What is an example of concatenation?
One of the most essential methods in JavaScript string formatting is concatenation. Concatenation is the process of appending one string to the end of another. The + operator, although commonly used for arithmetic, doubles as a tool for string concatenation in JavaScript. For instance, const fullName = "John" + " " + "Smith" would yield the complete string "John Smith".
Template literals
What are template literals? Are they better than concatenation?
Concatenation can become cumbersome for more complex strings or multi-line strings. This is where template literals come into play. Introduced in ES6, template literals are enclosed by backticks (` `) and allow for easier multi-line strings and string interpolation. In a template literal, placeholders denoted by the dollar sign and curly braces (${}) are replaced with their corresponding values. For instance, if we have const userName = 'John', we could use a template literal to format a greeting: const greeting = Hello, ${userName}! How are you?``.
Built-in methods
Strings in JavaScript also come with a host of built-in methods attached to the String.prototype object that provide additional functionality. This includes methods like toUpperCase(),toLowerCase(), and indexOf(). For example, const upperCaseGreeting = greeting.toUpperCase() would convert our previous greeting to all uppercase letters.
Advanced techniques
JavaScript's string formatting capabilities extend beyond just the basics. Advanced techniques involve:
- regular expressions (RegExp)
- substring method
- new String method
Regular expressions allow for powerful pattern matching and text manipulation, while the substring method extracts a part of a string and returns it as a new string. The new String method, on the other hand, creates a new string object - although, for performance reasons, it is generally recommended to use the string literal syntax instead.
What are template strings used for? Why are template literals important?
The following are some of the applications of template strings that explain their importance as well.
- Consider a scenario where we need to format a string dynamically with arguments (args) passed in a function call. Here, template strings shine by allowing us to embed expressions (which can be variables, function calls, or even complex calculations) seamlessly into a string.
- For instance, a date fetched from a new Date() function can be formatted and converted into a string using the toString() method, and then embedded into a template string for display.
- When interacting with CSS in a JavaScript environment such as jQuery, template strings can aid in dynamically setting styles.
- The flexibility of JavaScript even extends to conditional string formatting using the ternary operator, letting us choose between different string formats based on a condition. An example could be displaying a different greeting message based on the time of the day.
All these advanced techniques and more are showcased in numerous open-source projects on GitHub where you can learn and contribute as well.
String formatting in other programming languages
It's important to note that JavaScript is not the only programming language with string formatting capabilities. Python, for instance, has its string format method that uses the % operator. Similarly, Java employs the String.format function for string formatting.
Conclusion
In conclusion, understanding JavaScript string formatting is key for any web developer. From simple string concatenation to complex regular expressions, these tools empower developers to handle and manipulate text data efficiently and effectively. Happy coding!
Mastering JavaScript string formatting can be a challenging task, especially for young learners. Thankfully, YoungWonks offers premium quality coding classes for kids that provide a comprehensive and in-depth understanding of web development coding, including HTML, CSS and JavaScript. Moreover, for those interested in a more extensive course that covers broader aspects of web development, YoungWonks also provides Full Stack Web Development Classes. These classes delve into the realms of both front-end and back-end development, equipping students with all the necessary tools to become proficient web developers.
Here is a video to recap what we learned from the above blog.
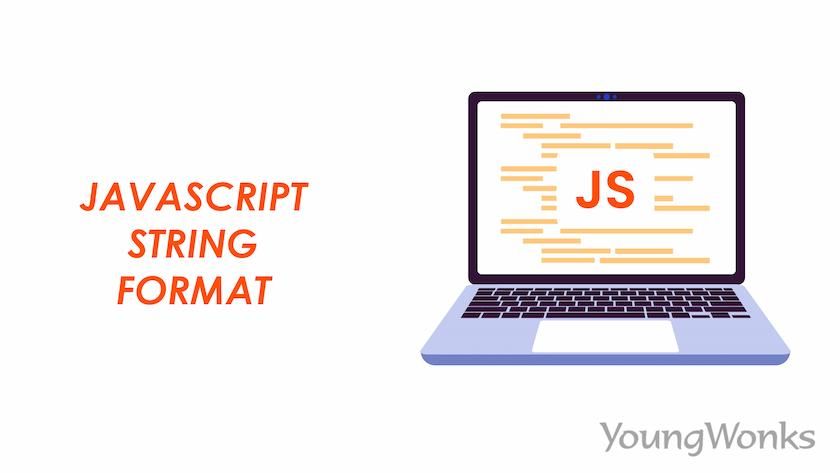
*Contributors: Written by Rohit Budania; Lead image by Shivendra Singh; YouTube video by Suchin Ravi